We’re going to see how to integrate an ER export format into a form. This involves adding a button that, when clicked, will execute a parameterized ER format and pass arguments from the currently selected record.
We have this table, where the first two strings act as a unique key:

Additionally, we have a Model, a Mapping, and an ER Format. The mapping has two user input parameters. These parameters are used to filter the record. Currently, when executing the format, the user must input these parameters manually. However, we aim for these to be filled automatically by the code.

First, let’s begin by adding a lookup to the ER table, enabling parameterization of which ER to execute. I typically incorporate this into a parameters form; for this example, we’ll add it to the ‘Accounts payable parameters’. In Visual Studio, we’ll extend both the ‘PurchParameters’ table and the ‘VendParameters’ form.

Next, we’ll drag the EDT ‘ERFormatMappingId’ into the ‘PurchParameters’ extension. This will automatically create the necessary relations. It’s a good idea to rename both the field and relation by adding a prefix to each and a clearer name:

Then we add the field anywhere we want (and where we can) on the form extension. To enable referencing it by code, change the ‘AutoDeclaration’ property to ‘Yes’.

And after compiling we get:

We can also filter this lookup to only display formats derived from a specific model. To do this, let’s copy the ‘OnLookup’ event handler of the field on the form and paste it into a new class.


Inside the method, let’s add the following:
ERFormatMappingTable::lookup(sender, "TST_TableMod", "Root");
FormControlCancelableSuperEventArgs cancelableArgs = e as FormControlCancelableSuperEventArgs;
cancelableArgs.CancelSuperCall();
The first line filters the lookup. To do this, we need to provide two string parameters, which can be found in our model:

The subsequent lines ensure that the super method won’t be called. Now, let’s compile. In our lookup, we’ll only see formats that extend from our model.

Now, we can add a button to the form. This button will trigger a class designed to execute the ER. First, let’s create a new class with the following code:

internal final class TST_ERRunner
{
static void main (Args _args)
{
TST_table001 tst_table001;
//We get the record from where we are calling our ER
select firstonly * from tst_table001 where tst_table001.RecId == _args.record().RecId;
//We pass the two parameters pointing to our mapping parameters
ERModelDefinitionInputParametersAction obj = new ERModelDefinitionInputParametersAction();
obj.addParameter('model/$String1', tst_table001.FieldString1);
obj.addParameter('model/$String2', tst_table001.FieldString2);
//We Run the ER, first parameter is the ER format, second is for overriding the format name
//The third is for showing the ER prompt
ERObjectsFactory::createFormatMappingRunByFormatMappingId(
PurchParameters::find().TST_ERFormatMappingIDForTSTTable,"",false).withParameter(obj).run();
}
}
Next, let’s create an ‘Action Menu Item’ that points to our class, and then drag it onto our form.


Let’s compile, and if everything has been set up correctly, our format should be exported with data from the currently selected record. In this case, it will be done directly, bypassing the usual ER prompt.
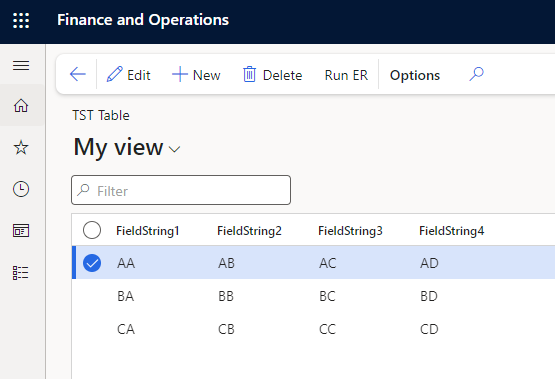
Leave a Reply